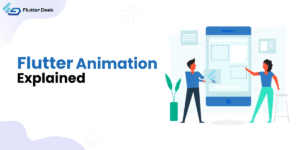
Flutter Animation Explained
Flutter is an incredibly robust and dynamic framework that empowers developers to craft visually stunning and captivating mobile applications. This framework stands out thanks to Flutter Animation’s exceptional capability to produce captivating animations that elevate the overall user experience. Flutter animation offers a comprehensive suite of resources for enhancing mobile application user experience (UX) by imbuing the user interface (UI) with seamlessness and authenticity. Let’s explore some fundamentals of Flutter animations with a Flutter animation tutorial. Flutter Animations-An Overview Using Flutter Animation, programmers can easily design interactive and aesthetically pleasing user interfaces. Animation in Flutter is like a visual illusion of motions made from images, widgets, or routes. To create an animation in Flutter, you define the initial and final states of an object or property and then tell it how to interpolate between them. Flutter Animation’s ease of use and adaptability are two of its most appealing qualities. It’s simple for developers to animate everything from simple buttons and icons to more complex widgets and snippets of text. The user experience can be fine-tuned by adjusting animation length, easing curves, and playback. Other Flutter features, such as gesture detection and user input, work harmoniously with Flutter Animation. What Are The Different Types Of Flutter Animations? Flutter has many animations to bring your app to life. Fade, scale, rotate, implicit, and explicit animations are among these. Understanding animation types will help you choose one for your app. Fade Animation flutter: This animation allows you to smoothly transition an element from being fully visible to completely invisible, or vice versa. Scale animation: This animation allows you to smoothly resize an element, making it appear larger or smaller. Rotate animation: This flutter animation allows you to rotate an element around a specified axis smoothly. It can create exciting effects, such as rotating a card when flipped or adding a playful touch to your app by animating a spinning logo. Implicit: Flutter’s implicit animations are the animated transitions that happen mechanically whenever a widget’s property is updated. If you want a seamless transition between a widget’s old and new values, you may use an implicit animation when you alter its color or size. Explicit: Explicit animations are preferred when you need to construct unique animations with fine-grained control over individual animation parameters, but they need more manual input. Common techniques include tweens, animation controllers, and animated widgets to manipulate the animation process directly. Flutter Animation Example When pressed, a button in your Flutter app should trigger an animation. Let’s make something exciting and valuable to showcase the potential of Flutter Animation. We want the button’s size to increase and decrease gradually, and its color will shift from gray to blue, then blue to grey when the user presses it. Flutter Animations can be used to create this effect. To begin, we establish a Flutter Animation Controller that manages the looping and stopping of the animation. To define the range of values for resizing and coloring, we’ll use a Tween. Once the button is pressed, we invoke the forward method of the AnimationController to begin the animation. We use Tween’s interpolated values to change the size and color of the button throughout the animation. import ‘package:flutter/material.dart’; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( title: ‘Animations’, theme: ThemeData( primarySwatch: Colors.purple, appBarTheme: const AppBarTheme(centerTitle: true), ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key}); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> with SingleTickerProviderStateMixin { late AnimationController _animationController; late Animation<double> _scaleAnimation; late Animation<Color?> _colorAnimation; @override void initState() { const duration = Duration(milliseconds: 500); _animationController = AnimationController(duration: duration, vsync: this); _scaleAnimation = Tween<double>( begin: 1.0, end: 2.0, ).animate(_animationController); _colorAnimation = ColorTween( begin: Colors.grey, end: Colors.blue, ).animate(_animationController); super.initState(); } @override void dispose() { _animationController.dispose(); super.dispose(); } Future<void> _onButtonPressed() async { await _animationController.forward(); await _animationController.reverse(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: const Text(‘Animations’)), body: Center( child: AnimatedBuilder( animation: _animationController, builder: (context, child) { return Transform.scale( scale: _scaleAnimation.value, child: ElevatedButton( style: ElevatedButton.styleFrom( backgroundColor: _colorAnimation.value, ), onPressed: _onButtonPressed, child: const Text(‘Like’), ), ); }, ), ), ); } } How To Make An Animated Widget In Flutter? Here is how you can add an animated widget in the Flutter application: Define a stateful Widget: Create a new class in your Dart file that inherits from StatefulWidget. Identify your stateful widget with this class. Define a new class in this file that inherits from the State class. This class will manage your widget’s state and logic. Define the animation: Set up an AnimationController inside the State class of your StatefulWidget. With this controller, you can change how long your animation lasts and how it plays. You can choose the length of time and other properties. Implement the animation logic: Override the initState() method inside the State class. Inside this method, you can set up the AnimationController and tell it how to move using Animation or Tween objects. You can choose from different animations, such as fading in, sliding, or scaling. Build the animated widget: You can use the AnimatedBuilder widget in your widget’s build() method to add animations. Return the widget you want to animate inside the builder function of AnimatedBuilder. Update the animation: The animation can be triggered by invoking forward(), reverse(), or repeat() on the animation controller. These methods can be called in response to input from the user or other events. import ‘package:flutter/material.dart’; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( title: ‘Animations’, theme: ThemeData( primarySwatch: Colors.purple, appBarTheme: const AppBarTheme(centerTitle: true), ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key}); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> with SingleTickerProviderStateMixin { late AnimationController _controller; late Animation<double> _scaleAnimation; @override void initState() { super.initState(); const duration = Duration(seconds: 2); _controller = AnimationController(duration: duration, vsync: this); _scaleAnimation = Tween<double>( begin: 1.0, end: 2.0, ).animate(_controller); _controller.repeat(); } @override void dispose() {